So you want to create a CFWheels application? ( Part 4)
This CFWheels series is heavily borrowed from Dan Wilson 's "So You Want to" series about Model Glue :Unity. This entry matches to this post . Previously in this series, we installed CFWheels , discussed some basic conventions and concepts in CFWheels, added our basic flow and navigation , and most...Published on
This CFWheels series is heavily borrowed from Dan Wilson's "So You Want to" series about Model Glue:Unity. This entry matches to this post. Previously in this series, we installed CFWheels, discussed some basic conventions and concepts in CFWheels, added our basic flow and navigation, and most recently created add and list functionality to our contacts. Our Contact-O-Matic is moving right along (this last sentence was borrowed from Dan's post.) In the last segment, we tested the 'success' path and were routed to the contact list. Now let's try the 'failure' path, add some validation, and refactor our code. Remember to get our form, we will use one of these URLs depending on the URL Rewriting you setup.
URL Rewriting On = http://localhost/contact/new
URL Rewriting Partial = http://localhost/index.cfm/contact/new
URL Rewriting Off = http://localhost/index.cfm?controller=contact&action=new
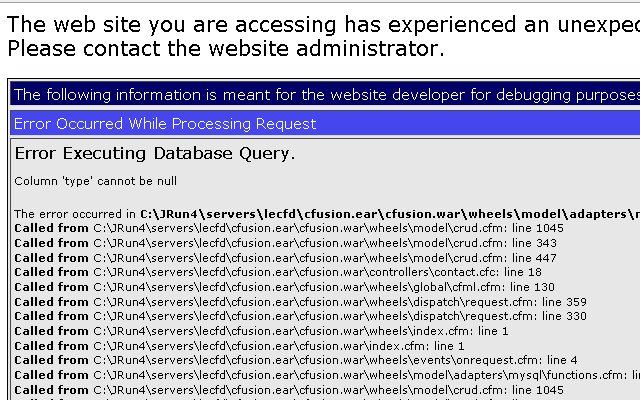
Validation
D'oh, so we need some validation apparently. Luckily for us, we are learning CFWheels which has built in validation to help us with saves, creates, and updates. In our model cfc of the contacts table, located at Models/contact.cfc, add this code:
<cffunction name="init">
<cfset validatesPresenceOf(property="name",message="Name is Required") />
<cfset validatesPresenceOf(property="type",message="Type of Contact is Required") />
<cfset validatesUniquenessOf(property="name", message="Name is already present") />
<cfset validatesInclusionOf(property="type",list="Friend,Enemy,Co-Worker",message="Type of Contact must be Friend, Enemy, or Co-Worker") />
</cffunction>
<cffunction name="create">
<cfset newContact = model("Contact").new(params.newContact)>
<cfif newContact.Save()>
<cfset flashInsert(success="User #params.newContact.name# created successfully.") />
<cfset redirectTo(action="list") />
<cfelse>
<cfset renderPage(action="new") />
</cfif>
</cffunction>
#flash("success")#
#errorMessagesFor("newContact")#
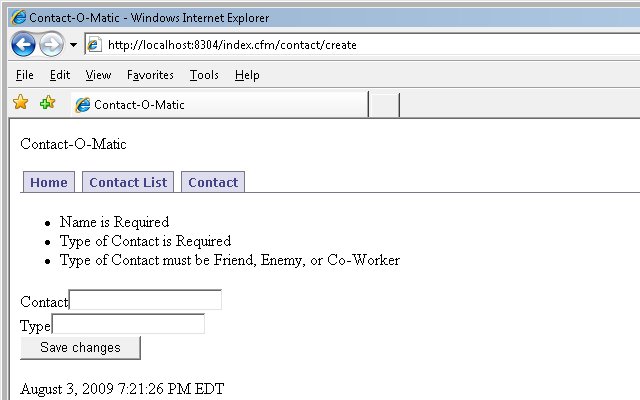
&reload=true
<cfset model("Contact").create(params.newContact)>
<cfset newContact = model("Contact").new(params.newContact)>
renderPage()
renderPage()
http://localhost/index.cfm/contact/create
renderPage()
Refactor
Now a little refactoring, you may remember we left our index action and view in a mess. Delete the index action in Controllers/contact.cfc and put this code in Views/contact/index.cfm .
<cfoutput>
<h1>Contact-O-Matic</h1>
This application is to manage contacts. It demonstrates how to build an application using <a href="http://www.cfwheels.org">CFWheels</a>
To add a contact #linkTo(text="click here",controller="contact",action="new")#.
To see a list of our contacts, #linkTo(text="click here",controller="contact",action="list")#.
</cfoutput>
URL Rewriting On = http://localhost/contact/
URL Rewriting Partial = http://localhost/index.cfm/contact
URL Rewriting Off = http://localhost/index.cfm?controller=contact&action=index
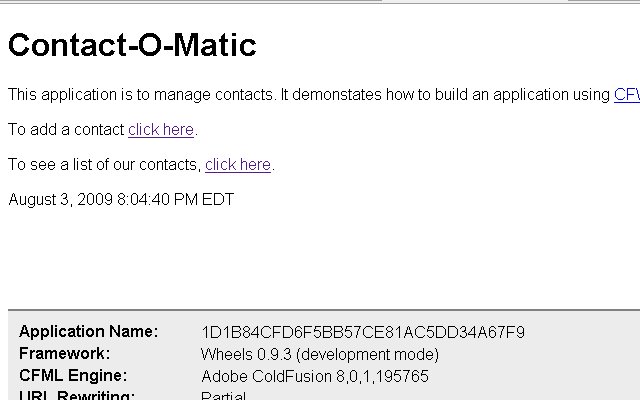
linkto()
More Refactoring
You may have noticed our navigation wasn't working, we created a Partial called _Banner.cfm and placed it in the Views/contact/ folder. Replace the current code with this
<cfoutput>
<div id="banner">
Contact-O-Matic
<ul id="navlist">
<li <cfif params.action IS "home">id="current"</cfif> >#linkTo(text="Home",controller="contact",action="index")#</li>
<li <cfif params.action IS "list">id="current"</cfif>>#linkTo(text="Contact List",controller="contact",action="list")#</li>
<li <cfif ListContains("new,create", params.action)>id="current"</cfif> >#linkTo(text="Contact",controller="contact",action="new")#</li>
</ul>
</div>
</cfoutput>
#navlist li a
{
background: white;
border-bottom: 1px solid white;
}
#navlist #current a
{
background: white;
border-bottom: 1px solid white;
}
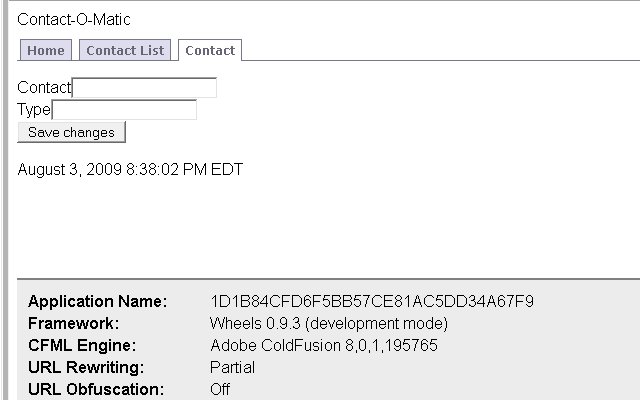